숙소 리뷰를 다음과 같은 조건으로 만든다.
1) 가장 첫 칸에는 자신의 리뷰를 출력한다.(sessionId와 리뷰의 id 컬럼 비교)
2) 그 뒤로 출력되는 리뷰 목록에서는 자신의 리뷰를 제외한다(위와 마찬가지)
리뷰 테이블은 hotel_review, spot_review 등이 있다.
리뷰 테이블은 다음과 같이 구성된다.
num: serial PK
contentSeq: int FK
text: text
mem_id: varchar
rev_date: timestamp default current_timestamp
컨트롤러의 메서드는 5가지 부분으로 나뉜다.
1) 숙소 상세정보 출력
2) 리뷰 리스트 획득, model에 추가
3) 세션 아이디와 같은 아이디로 작성된 리뷰가 있는지 확인
4) 있다면 가져와서 model에 따로 추가
5) 페이지 리턴
이 포스트에서는 3번과 4번만 진행한다.
@GetMapping("/hotel") //상세보기용
public String requestHotelByNum(@RequestParam("num") String contentSeq, Model model, @ModelAttribute("NewReview") Review review)
{
//1)
System.out.println("HotelController.requestHotelByNum() 도착");
Hotel hotelByNum = hotelService.getHotelByNum(contentSeq);
model.addAttribute("hotel", hotelByNum);
//2)
//리뷰
List<Review> list = reviewService.getAllReviewList(contentSeq, "hotel");
model.addAttribute("reviewList", list);
//3)
String sessionId = (String)session.getAttribute("sessionId");
System.out.println("sessionId: " + sessionId);
int verify = reviewService.getCountReviewByIdAndContentSeq(sessionId, contentSeq);
model.addAttribute("verify", verify);
//4)
if(verify == 1) {
System.out.println("리뷰 있음");
Review myReview = reviewService.getReviewByIdAndContentSeq(sessionId, contentSeq, "hotel");
System.out.println("myReview text: " + myReview.getText());
model.addAttribute("myReview", myReview);
}
//5)
return "hotel/hotel";
}
3) 세션 아이디와 같은 아이디로 작성된 리뷰가 있는지 확인
(1)컨트롤러
String sessionId = (String)session.getAttribute("sessionId");
System.out.println("sessionId: " + sessionId);
int verify = reviewService.getCountReviewByIdAndContentSeq(sessionId, contentSeq);
model.addAttribute("verify", verify);
(2)리파지토리(서비스 생략)
컨트롤러가 실행될 때 파라미터로 받은 contentSeq와 세션의 sessionId를 사용해서 데이터베이스를 조회한다. verify가 1이라면 이 아이디에서 작성된 리뷰가 존재한다.
public int getCountReviewByIdAndContentSeq(String sessionId, String contentSeq) {
System.out.println("reviewRepository.getCountReview() 도착");
System.out.println("sessionId: " + sessionId);
System.out.println("contentSeq: " + contentSeq);
String sql = "SELECT COUNT(*) FROM hotel_review WHERE mem_id = ? AND contentSeq = ?";
int verify = template.queryForObject(sql, Integer.class, sessionId, contentSeq);
return verify;
}
4) 있다면 가져와서 model에 따로 추가
(1)컨트롤러
if(verify == 1) {
System.out.println("리뷰 있음");
Review myReview = reviewService.getReviewByIdAndContentSeq(sessionId, contentSeq, "hotel");
System.out.println("myReview text: " + myReview.getText());
model.addAttribute("myReview", myReview);
}
(2)리파지토리(서비스 생략)
public int getCountReviewByIdAndContentSeq(String sessionId, String contentSeq, String category) {
System.out.println("reviewRepository.getCountReview() 도착");
System.out.println("sessionId: " + sessionId);
System.out.println("contentSeq: " + contentSeq);
String sql = "SELECT COUNT(*) FROM " + category + "_review WHERE mem_id = ? AND contentSeq = ?";
int verify = template.queryForObject(sql, Integer.class, sessionId, contentSeq);
System.out.println("verify: " + verify);
return verify;
}
출력 뷰
1) 가장 첫 칸에는 자신의 리뷰 출력
스크립틀릿 태그 사용
<%
int verify = (int)request.getAttribute("verify");
if(verify == 1){
%>
<div id="리뷰" class="p-5" style="width: 32%; margin-right: 1%; border-radius: 10px; height: 350px; padding-top: 5px !important;">
<p style="margin-bottom: 5px">나의 리뷰</p>
<form action="review/update" method="POST" class="form-horizontal">
<textarea name="text" class="star_box" placeholder="리뷰 내용을 작성해주세요. (최대 200자)" maxlength="200">${myReview.text}</textarea>
<input type="hidden" name="mem_id" value="${myReview.mem_id}"/>
<input type="hidden" name="contentSeq" value="${myReview.contentSeq}"/>
<input type="submit" class="submit-green" value="리뷰 수정"/>
<a href="review/delete?num=${myReview.num}&contentSeq=${myReview.contentSeq}" class="submit-red" onclick="return confirm('리뷰를 삭제하시겠습니까?');">리뷰 삭제</a>
</form>
</div>
<%
}else{
%>
<div id="리뷰" class="p-5" style="width: 32%; margin-right: 1%; border-radius: 10px; height: 350px; padding-top: 5px !important;">
<p style="margin-bottom: 5px">리뷰 작성</p>
<form:form modelAttribute="NewReview" class="form-horizontal">
<form:textarea path="text" class="star_box" placeholder="리뷰 내용을 작성해주세요. (최대 200자)" maxlength="200" />
<input type="submit" class="btn02" value="리뷰 등록"/>
</form:form>
</div>
<%
}
%>
2) 그 뒤로 출력되는 리뷰 목록에서는 자신의 리뷰 제외
<c:if> 사용
<div class="d-flex" style="width: 67%">
<c:forEach items="${reviewList}" var="review">
<c:if test="${review.mem_id != sessionScope.sessionId}">
<div id="printReview" class="p-5" style="padding: 5px 50px 50px!important; height: 400px; width: 49%; ">
<p style="margin-bottom: 5px">${review.mem_nickname} (${review.rev_date})</p>
<div class="star_box">
<p>${review.text}</p>
</div>
<div style="margin-bottom: 100px">
<!-- 로그인 했을 때만, 본인 리뷰 아닐 때 -->
<a href="" style="text-decoration: none; color: gray" class="me-1"><i class="fas fa-exclamation-circle me-1"></i>리뷰 신고</a>
</div>
</div>
</c:if>
</c:forEach>
</div>
결과
내가 작성한 리뷰가 없는 경우
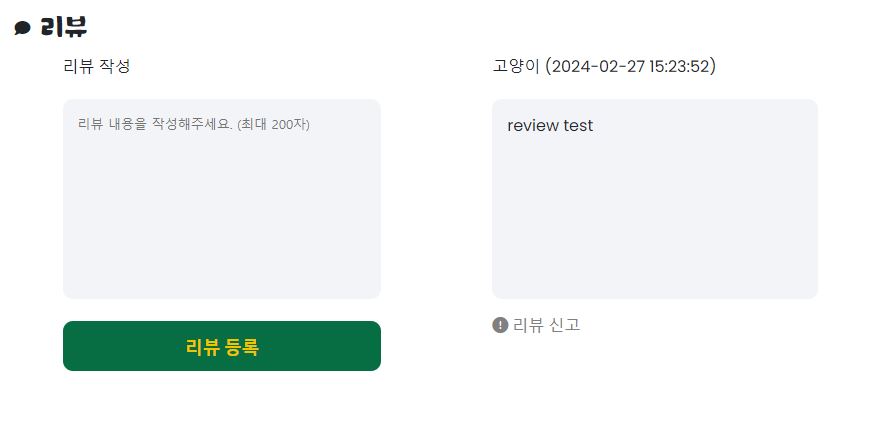
내가 작성한 리뷰가 있는 경우
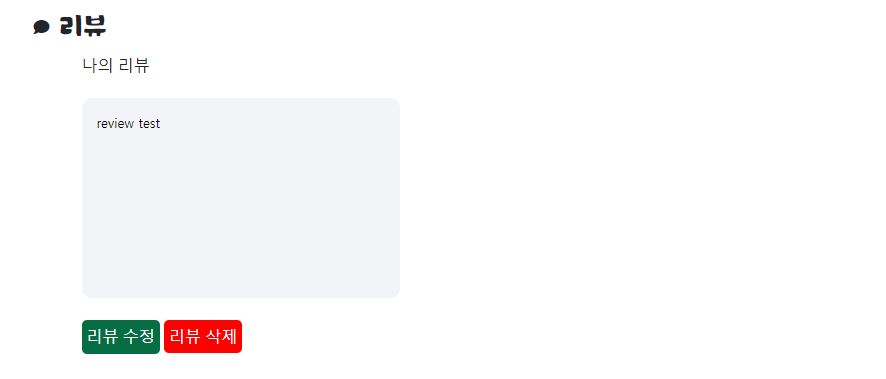
'정리노트 > 팀프로젝트' 카테고리의 다른 글
[팀 프로젝트] 24.02.28. 노트 (0) | 2024.02.28 |
---|---|
[JS]button 클릭시 엉뚱한 폼이 제출되는 문제: preventEvent() (0) | 2024.02.28 |
[팀 프로젝트] 24.02.27. 노트 (0) | 2024.02.27 |
[팀 프로젝트] 24.02.26. 노트 (0) | 2024.02.26 |
[팀 프로젝트] 24.02.22. 노트 (0) | 2024.02.22 |